Get coupon code API
Get Coupon Code API enables users to retrieve coupon code data based on country.
Getting Started:
By using the Get Coupon Code API, users gain access to the coupon code specific to the country code which is passed in the API. If the user can retrieve the country location of their website visitors, this API assists in verifying whether the website visitor has utilized the coupon code corresponding to their country before proceeding with transactions.
Get coupon code API
https://api.paritydeals.com/api/v1/deals/country/discount/?pd_identifier=BANNER_IDENTIFIER&country_code=IN
Sample code
const apiKey = 'YOUR_API_KEY';
async function getCouponCode() {
const response = await fetch(
'https://api.paritydeals.com/api/v1/deals/country/discount
/?pd_identifier=BANNER_IDENTIFIER&country_code=IN',
{
headers: {
Authorization: `Bearer ${apiKey}`,
},
}
);
if (!response.ok) {
throw new Error('Failed to fetch coupon code');
}
return await response.json();
}
async function fetchCoupon() {
try {
const couponResponse = await getCouponCode();
console.log(couponResponse);
} catch (error) {
console.error('Error fetching coupon code:', error);
}
}
fetchCoupon();
How it works
Base URL
https://api.paritydeals.com/api/v1/deals/country/discount/
This is the base URL of the API.
Query Parameters
pd_identifier: This parameter is used to specify a unique identifier associated with a particular deal. You need to replace ‘BANNER_IDENTIFIER’ with the actual identifier you want to use.
country_code: This parameter is used to specify the country for which the you wants to fetch coupon code. You need to replace ‘IN’ with the country code that you want to check.
You will be then required to make an HTTP GET request on your website. Pass the ParityDeals API key in the header of the HTTP GET request using the following format:
headers: {
Authorization: `Bearer ${apiKey}`,
}
Replace ${apiKey} with your actual ParityDeals API key.
How to get the banner identifier?
Every banner has a secret identifier associated with it.
You can access the banner identifier from
How to get the ParityDeals API key?
- Step 1 :
Click on the user avatar on the home page and select the “Settings” option from the dropdown menu.
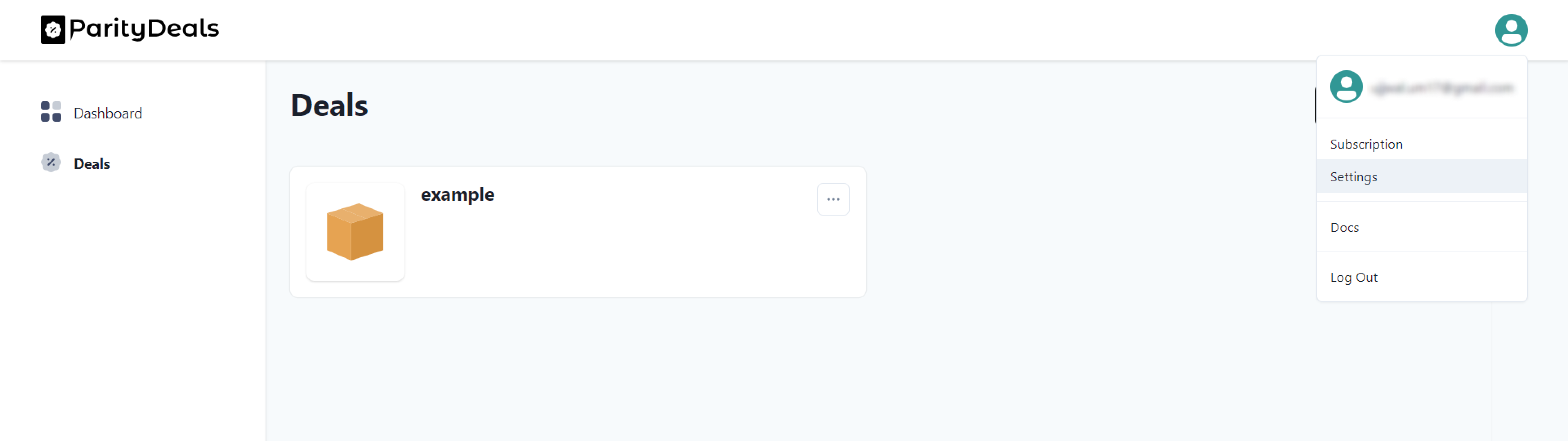
- step 2 :
Navigate to the “API Key” tab and click on the Create API Key button to generate your API key.
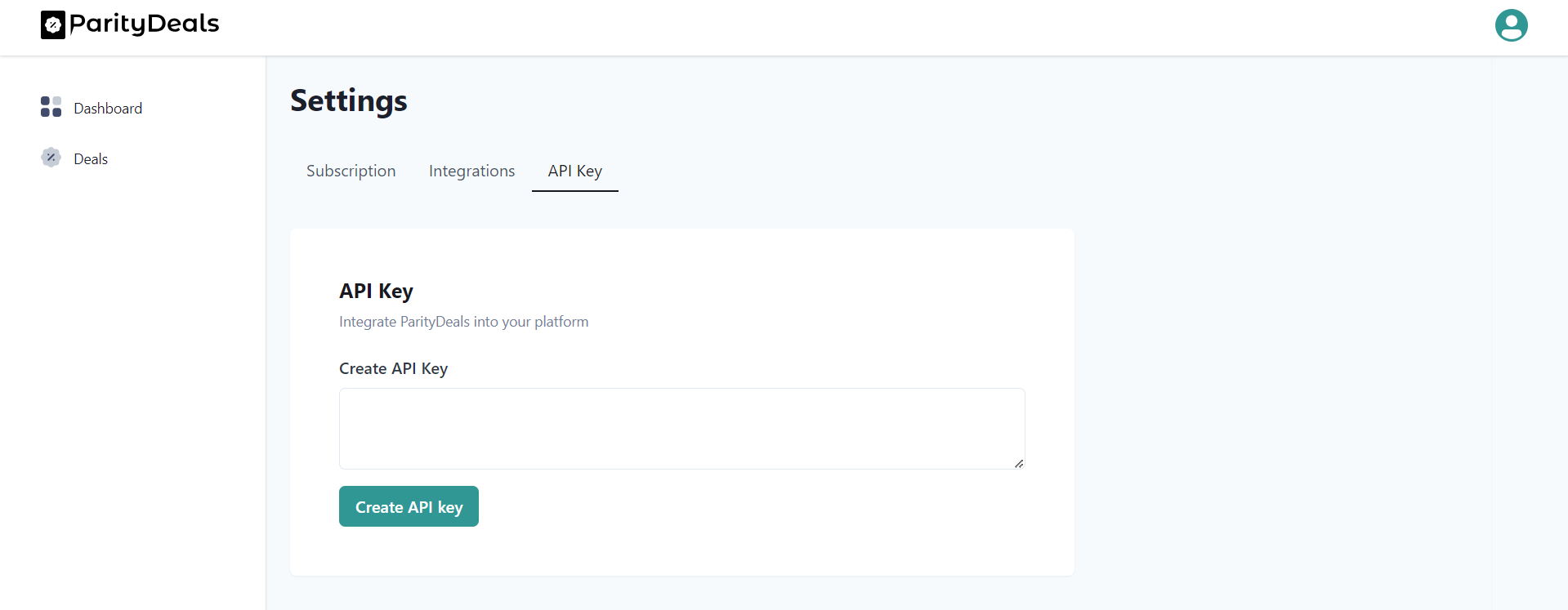